In the expansive and nuanced landscape of C programming, developers often find themselves at the crossroads of a pivotal decision – choosing between string
and cstring
. This exhaustive exploration aims to plunge into the depths of these essential concepts, unraveling their subtle distinctions, exploring diverse use cases, and discerning their potential impact on C programming endeavors. As we embark on this extensive journey, our goal is to comprehensively dissect the string vs cstring in C debate, shedding light on the intricacies that encompass these fundamental aspects. By the end of this expedition, developers will be equipped with a profound understanding of character sequences in C, enabling them to make informed choices tailored to the specific needs of their projects.
Table of Contents
Understanding Strings in C
The Basics:
In the foundational realm of C, the concept of strings revolves around an array of characters terminated by a null character ('\0'
). At the heart of this abstraction lies the string
type, offering developers a powerful tool for handling character sequences. This section aims to delve deeper into the fundamental aspects of strings, exploring their inherent structure and introducing developers to the diverse ways they can be initialized and manipulated.
Usage and Functions:
- Declaration and Initialization:
- Strings, as encapsulated by the
string
type, are declared using thechar
array syntax. Initialization is a flexible process that can occur during declaration or later through standard assignment. Developers will find the nuances of this process, providing insights into crafting efficient and readable code.
- Strings, as encapsulated by the
cCopy code
char myString[10] = "Hello";
- Common Functions:
- The repertoire of standard library functions available for string manipulation is extensive. Functions such as
strlen()
,strcpy()
,strcat()
, andstrcmp()
are invaluable tools that form the backbone of string operations. This section will explore these functions in detail, unraveling their capabilities and guiding developers on their optimal usage.
- The repertoire of standard library functions available for string manipulation is extensive. Functions such as
cCopy code
#include <string.h> int length = strlen(myString);
Exploring Cstrings
What is a Cstring?
The term “Cstring” is frequently employed interchangeably with “C-style string.” In essence, a Cstring in C programming is a null-terminated array of characters, akin to the broader concept of strings. This section endeavors to provide a profound understanding of Cstrings, elucidating their primitive nature and contrasting it with the higher-level abstractions presented by the string
type.
Usage and Functions:
- Declaration and Initialization:
- Similar to strings, Cstrings are declared using the
char
array syntax. The process of initialization mirrors that of strings, but with distinct nuances. This section will guide developers through the intricacies of declaring and initializing Cstrings, offering insights into best practices.
- Similar to strings, Cstrings are declared using the
cCopy code
char myCstring[10] = "Hello";
- Common Functions:
- Cstrings leverage standard library functions such as
strlen()
,strcpy()
,strcat()
, andstrcmp()
for basic yet powerful character sequence manipulations. This section will dive into the application of these functions in the context of Cstrings, providing developers with a comprehensive toolkit.
- Cstrings leverage standard library functions such as
cCopy code
#include <cstring> int length = strlen(myCstring);
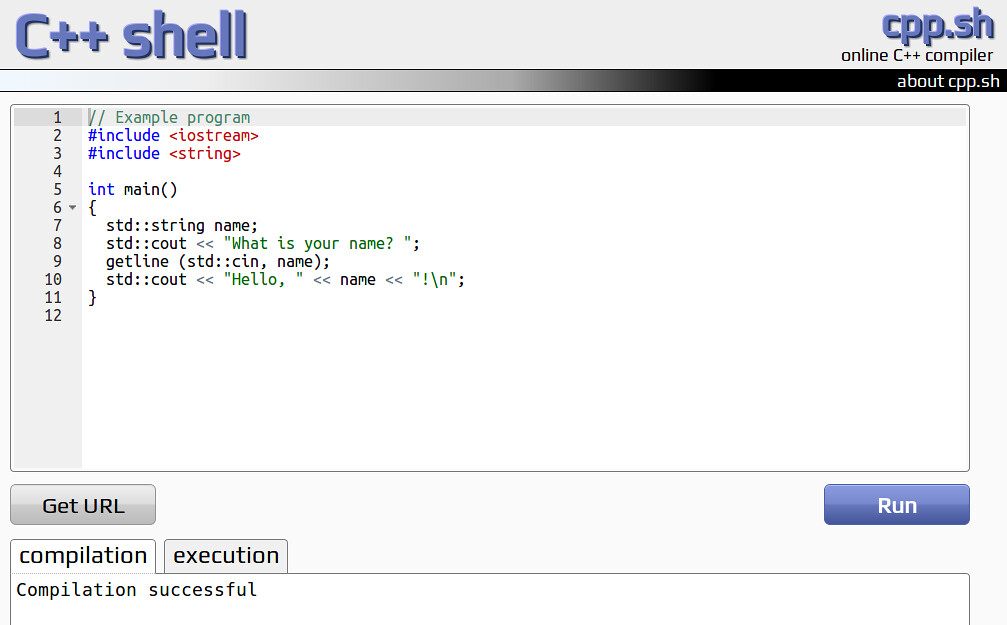
String vs Cstring in C: Unraveling the Differences
1. Abstraction Level Comparison Between String VS CString in C:
- String:
- The
string
type boasts a higher-level abstraction, simplifying code with built-in safety features. This section will explore the convenience and expressiveness offered by thestring
type, shedding light on how it elevates the developer experience.
- The
- Cstring:
- Operating at a lower level of abstraction, Cstrings demand manual handling and null termination for proper functionality. This section will delve into the intricacies of low-level operations, empowering developers with a nuanced understanding of Cstrings.
2. Mutability Comparison Between String VS CString in C:
- String:
- Strings, encapsulated by the
string
type, are generally immutable. Modifications to a string result in the creation of a new string. This section will explore the implications of immutability, offering insights into the trade-offs and benefits.
- Strings, encapsulated by the
- Cstring:
- Cstrings exhibit mutability, allowing developers to modify individual characters directly. This section will guide developers through the process of leveraging this mutability, emphasizing the fine-grained control it affords.
3. Library Dependence Comparison Between String VS CString in C:
- String:
- The
string
type relies on the Standard C++ library (#include <string>
). This section will unravel the dependencies and advantages associated with using the C++ standard library in the context of strings.
- The
- Cstring:
- Cstrings, on the other hand, utilize the Standard C library (
#include <cstring>
), maintaining compatibility with traditional C programming. This section will delve into the implications of this library choice, providing developers with insights into the historical and practical aspects.
- Cstrings, on the other hand, utilize the Standard C library (
4. Null Termination Comparison Between String VS CString in C:
- String:
- Strings, owing to the higher-level abstractions of the
string
type, automate null termination, simplifying operations and enhancing safety. This section will elucidate the significance of null termination, emphasizing its role in ensuring proper functionality.
- Strings, owing to the higher-level abstractions of the
- Cstring:
- Cstrings, being more primitive, mandate explicit null termination for proper functionality. This section will guide developers through the process of managing null termination manually, providing a comprehensive understanding of this critical aspect.
5. Performance Considerations Comparison Between String VS CString in C:
- String:
- While the performance difference between strings and Cstrings is typically negligible, the higher-level abstractions might introduce slight overhead. This section will explore the nuances of performance considerations associated with using the
string
type.
- While the performance difference between strings and Cstrings is typically negligible, the higher-level abstractions might introduce slight overhead. This section will explore the nuances of performance considerations associated with using the
- Cstring:
- Cstrings, with their lower-level nature, may offer a marginal performance advantage in specific use cases. This section will delve into scenarios where this advantage becomes pronounced, aiding developers in making informed performance-centric decisions.
Pros and Cons
Pros of Using String:
- High-level abstractions simplify code, enhancing readability and expressiveness. This section will provide examples and case studies showcasing the benefits of using the
string
type in different contexts. - Safer, reducing common programming errors. Developers will gain insights into how the
string
type mitigates potential pitfalls, fostering a secure coding environment. - Rich set of member functions for manipulation. The vast array of member functions available to strings will be explored, showcasing their versatility in various scenarios.
Cons of Using String:
- Potential overhead due to abstraction. This section will delve into the potential performance costs associated with using the
string
type, providing guidance on mitigating such overhead. - Limited direct control over individual characters. Developers will understand the trade-off between abstraction and control, gaining insights into scenarios where direct character manipulation is essential.
Pros of Using Cstring:
- Direct control over individual characters. The section will provide detailed examples of scenarios where direct control over characters becomes paramount, showcasing the advantages of Cstrings in specific use cases.
- Lower-level operations allow for more fine-grained manipulation. Developers will gain an understanding of the fine-grained control afforded by Cstrings, exploring scenarios where such operations are indispensable.
- Can be more memory-efficient for specific use cases. This section will delve into memory efficiency considerations, providing developers with insights into scenarios where Cstrings present an advantage.
Cons of Using Cstring:
- Prone to buffer overflows and other low-level errors. Developers will learn about common pitfalls associated with Cstrings and strategies for avoiding buffer overflows and related errors.
- Requires manual null termination for proper functionality. The importance of explicit null termination will be emphasized, with developers gaining a thorough understanding of how to manage this aspect effectively.
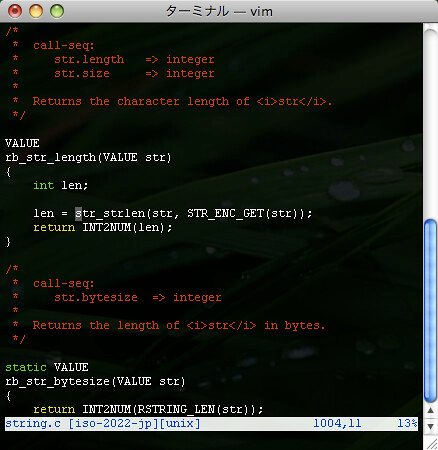
FAQ Section:
1. Can I mix string and Cstring operations in the same program?
Yes, developers can seamlessly integrate string and Cstring operations within the same program. However, this section will provide detailed insights into potential challenges and best practices for ensuring compatibility and avoiding unexpected behavior.
2. What precautions should I take when working with Cstrings to avoid common errors?
Working with Cstrings demands a set of precautions to avoid common errors. This section will delve into best practices, offering guidance on ensuring proper null termination, using functions like strcpy_s
for bounds checking, and exercising caution during manual manipulations.
3. Can I modify individual characters in a string?
No, strings are generally immutable in C++. This section will explore the implications of immutability, providing clarity on why modifications to a string result in the creation of a new string. Developers will also gain insights into scenarios where mutability becomes crucial.
4. Are there specific scenarios where Cstrings are more suitable?
Cstrings find their niche in specific scenarios where low-level control over memory and individual characters is crucial. This section will provide detailed examples of such scenarios, aiding developers in recognizing when Cstrings offer a distinct advantage.
5. Are there any best practices for choosing between string and Cstring?
Choosing between string
and cstring
involves considering various factors. This section will delve into best practices, guiding developers on factors such as the required level of abstraction, safety considerations, and the nature of operations they intend to perform. Developers will gain a comprehensive framework for making informed choices.
6. Can I dynamically allocate memory for strings and Cstrings?
Yes, both strings and Cstrings can be dynamically allocated in C programming. However, developers must exercise caution to avoid memory leaks and properly manage the allocated memory. This section will provide a detailed guide on dynamic memory allocation for both strings and Cstrings.
7. How do strings and Cstrings handle concatenation differently?
Strings and Cstrings handle concatenation differently, reflecting their underlying abstractions. This section will explore the distinctive approaches to concatenation, providing insights into the mechanisms employed by the string
type and Cstrings. Developers will gain a nuanced understanding of how each handles this fundamental operation.
8. Can I use string functions on Cstring literals and vice versa?
While string functions can often be used with Cstring literals and vice versa, developers must exercise caution. Cstring literals are treated as constants, and attempting to modify them directly can result in undefined behavior. For Cstrings, proper null termination is crucial. This section will delve into the intricacies of using string functions across both types, providing guidance on ensuring consistency and avoiding potential pitfalls.
9. How does the choice between string vs Cstring in C impact code readability?
The choice between string
and cstring
can significantly impact code readability. This section will explore the readability implications of each choice, providing insights into scenarios where the higher-level abstractions of the string
type contribute to more expressive and comprehensible code.
10. Are there specific considerations for using strings and Cstrings in multi-threaded applications?
In multi-threaded applications, proper synchronization mechanisms are crucial when working with both string vs Cstring in C. This section will provide a comprehensive guide on considerations for multi-threaded programming, addressing issues such as race conditions and data corruption during concurrent read and write operations on shared string data.
Conclusion:
The intricate decision between string
vs cstring
in C programming encompasses a myriad of factors, each playing a vital role in determining the efficacy and safety of code. This section will provide a reflective summary, encapsulating the key takeaways from the entire exploration. Developers will gain a holistic understanding of the dynamic interplay between strings and Cstrings, empowering them to make informed decisions that align with the specific needs of their projects. The profound insights garnered throughout this extensive exploration will serve as a valuable compass in the intricate landscape of character sequence manipulation in C programming. (If you’re interested in computer programming, do check out our post on the comparison of semaphores and mutex locks.)